Ideas are the building blocks of code. They give you the scaffold of how to think, how to create, and how to best write your code. Without it, you’ll be coding yourself into a corner in no time.
Lack of knowledge and awareness is also how code smell starts. Here are three major and foundational ideas in JavaScript that you should know about if you want to upgrade from a slightly confused junior developer to pro skilled engineer.
Scope
Everyone knows what a scope is but not at the same time. In short, think of it like a ring-fence that gives accessibility to your variables.
Here’s a diagram:
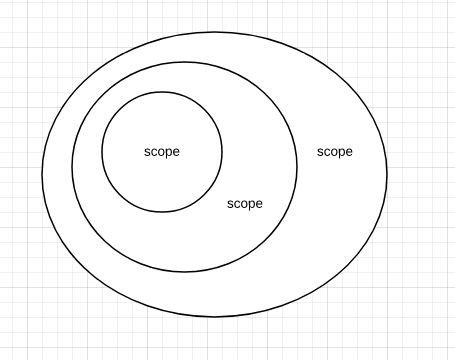
There are two types of scopes — a local scope and a global scope.
A local scope is one where it is only accessible by a particular “ring-fence.” In the image above, the innermost scope is a local scope. This is because it’s accessible within the context of that ring-fence.
Accessibility of scope trickles inwards. This means that scopes are not accessible beyond their boundary lines but can be used by those within.
The two inner rings of scopes in the image above can use the other ring variables but not the other way around.
A global scope is a variable where all scripts and functions have access to it. There is no ring-fence as such around a global scope, making it highly accessible.
Quick notes on let and var
let
and var
are ways to declare a variable in JavaScript. Although they both do sort of the same things, there are some differences between the two.
let
is only available inside the scope where it’s declared. var
defines a variable globally, or locally to an entire function regardless of block scope.
Think of a block scope as where your { }
pair starts and ends. A loop, for example, is one block scope. let
limits accessibility to block scope while var
limits accessibility to function scope.
Where you declare matters
You can create a global scope in JavaScript by using either let
or var
keyword before your variable name.
var catName = "Tibbers";
let dogName = "Roffles";
function callCat(){
console.log("Here, here, " + catName);
}
function callDog(){
console.log("Here, here, " + dogName);
}
When you declare your variables at the top-most level, it becomes a global scope.
Alternatively, you can attach it to the window
object. The window
object is an object that var
attaches to when a variable name is declared using it.
So, writing something like this will also work:
window.cat = "meow";
console.log(cat);
The Conditionals
if else
statements can be fun and easy to write — until they get a bit out of hand.
Alternatives you can use are:
Switch
A condition is based on a series of cases. If the condition fits, the relevant case is selected and executed.
Here’s what it looks like:
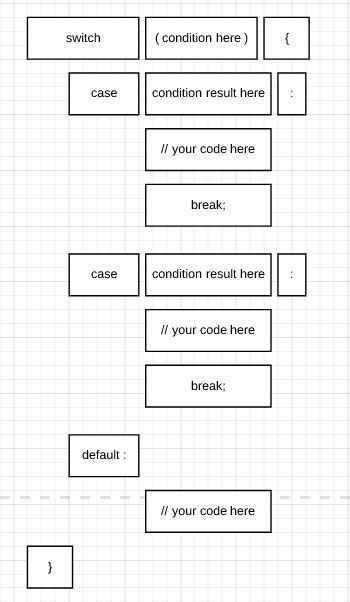
In code form, it looks something like this:
switch (catName){
case "Tibbers":
console.log("Yes! I am a cat!");
break;
case "Roffles":
console.log("I'm a dog! Don't be silly.");
break;
default:
console.log("quack quack. What am I?");
}
The break
keyword tells the JavaScript interpreter to stop running the code. switch
is good to use when you are faced with the possibility of multiple outcomes and the code to handle is not that large.
Alternatively, you can execute other functions based on the case’s outcome.
Loops
Loops can get your head a bit loopy if you’re not clear on the syntax. Here’s a diagram to help break it down for you:
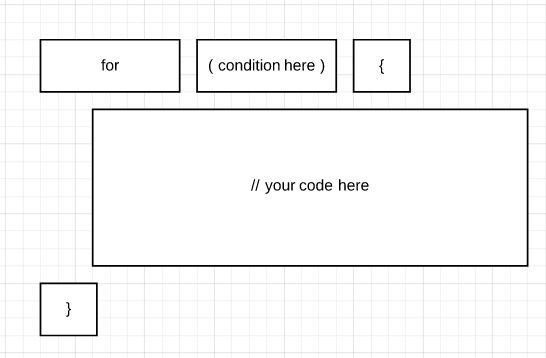
There are five different kinds of loops — for
, for/in
, for/of
, while
, and do/while
.
for loops
A for
loop has three statements in the condition area.
- The first statement is what gets executed before the code runs. This part gets executed once only.
- The second statement is the condition for executing the code block.
- The third statement is executed after your code gets executed.
In code, it looks something like this:
for (statement 1; statement 2; statement3){
// some code here
}
Here’s what it looks like in diagram form:
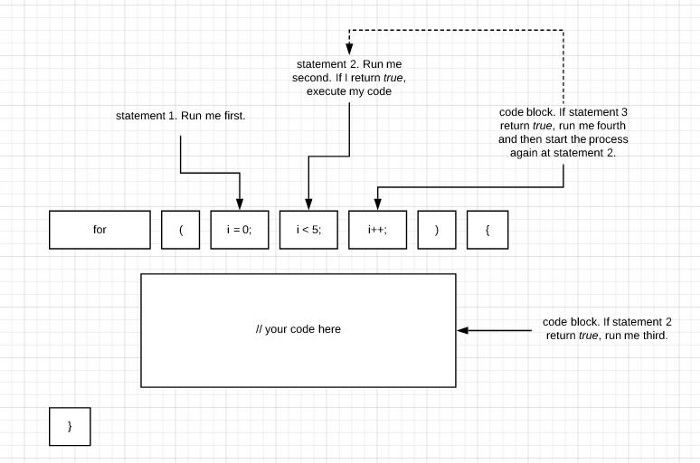
for/in loops
Let’s pretend you have an object. And, for whatever reason, you need to work your way through the properties of this object.
Never fear! for/in
loop is here!
How it works:
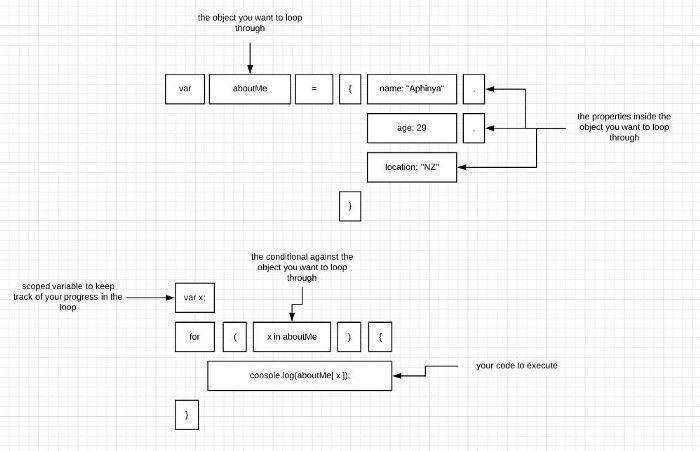
What it looks like in code:
var yourObjNameHere = { name: "Tibbers", type: "cat", color: "ginger" };
var loopMe;
for( loopMe in yourObjNameHere ) {
//do something with yourObjNameHere[loopMe]
}
for/of loops
for of
statements loop through the values of iterable objects. So, this means you can use it on arrays, strings, maps, and anything that looks like a list of some sort.
What it looks like:
var yourIterable = ["Tibbers", "Ruffles", "Rufus", "Roland"];
var trackerVariable;
for ( trackerVariable of yourIterable ) {
//do something with trackerVariable
}
for of
statements can also loop over strings. This means that it’ll go through each character of your string and the trackerVariable
is representative of the position and value.
Example:
var someTxt = 'Hello there!';
var x; //aka, your tracker variable
for( x in someTxt ){
console.log(x);
}
while
The while
loop continues to execute a block of code as long as the condition remains true
. This means that the condition has to evaluate to a boolean
result.
Rather than using for
, the keyword while
is used instead.
In code, it looks something like this:
while( condition ){
//some code here
}
If you are iterating over something for a specified amount of time, you’ll need to be clear on this too.
For example:
var i;
while( i < 5 ){
//do something
i++
}
In the example above, the i
needs to be increased, otherwise, the loop will continue to run until the end of time (or when your code crashes).
do/while
do while
is a similar concept to while
, just written differently. The difference between do while
and while
is that do while
will execute at least once, even if the condition is false
.
Here’s the syntax for it:
do {
//what am I doing? you tell me...
} while (condition)
Promises
A promise happens when your code needs to be halted momentarily as it does something. This can happen when you’re waiting for a result from an API or there’s a delay for whatever reason.
The thing with JavaScript code is that it will charge on, from top to bottom, without waiting. In part, it’s because it doesn’t know when to wait.
That’s what a promise does. It tells your code to hold up, wait for a response, and then move on with whatever it was supposed to be doing.
There are three parts to a promise — the evocation, the wrapper, and the returned result.
Here’s what it looks like in diagram form:
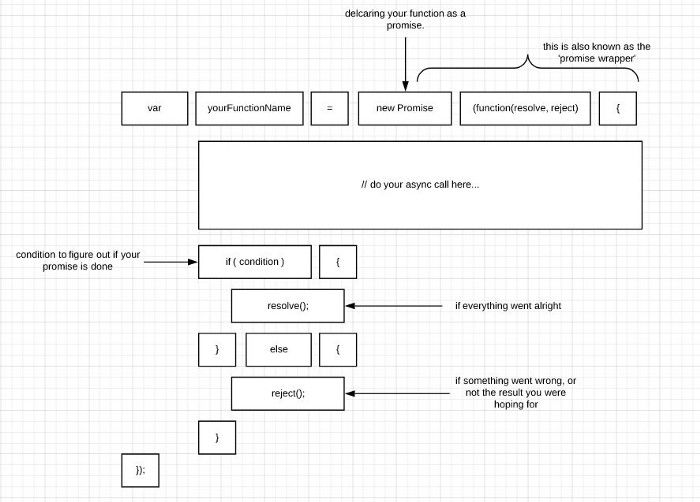
To use a promise, you tag .then()
onto the end of your called promise function. Whatever gets resolved in resolve()
is passed through to then()
.
In code, it looks something like this:
yourPromisedFunction().then((resolvedObj) => {
//do something with resolvedObj
});
There’s more to promises but that’s the main part of it in a nutshell.
Final Words
There are plenty more concepts in JavaScript that are important but these are the three that I often find myself explaining to help others improve their syntax and code structures.
Yes. They are foundational ideas.
And yes, I have simplified them for ease of mental digestion.
If you’re a beginner, I hope you’ve found this useful and thank you for reading.