5 JavaScript Refactoring Patterns That Actually Scale
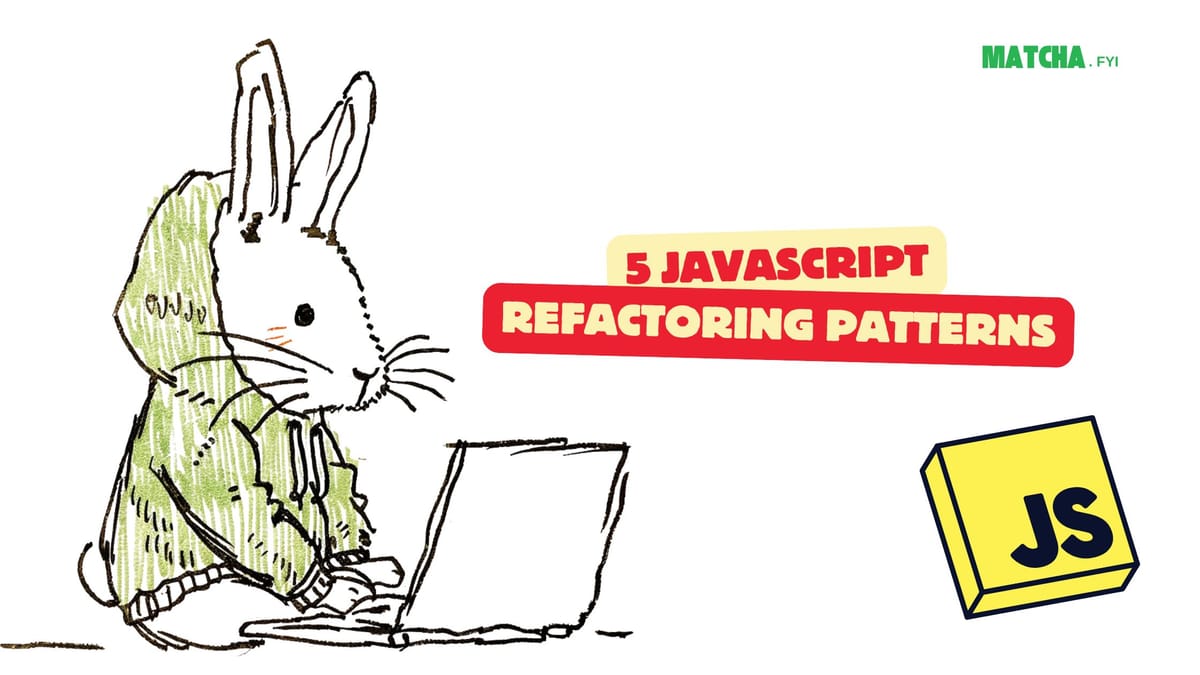
Refactoring isn’t optional. It’s mandatory if you’re building a product with any ambition to scale.
Most codebases rot because they don’t scale—features get bolted on, tests break, and nobody wants to touch critical parts of the system without praying first.
You don’t fix that with prettier functions. You fix it with patterns that hold the line when the team, the feature set, and the customer base all double.
This post gives you 5 refactoring patterns that scale. They’re tested in production. They’ll keep your JavaScript codebase healthy as it grows.
1. Dependency Injection (DI): Eliminate Hidden Coupling
Hardcoding dependencies guarantees pain.
You want loose coupling with explicit dependencies.
The Problem
This is bad:
function fetchUser(userId) {
return axios.get(`/users/${userId}`);
}
- You can’t swap
axios
forfetch
or anything else. - You can’t test this in isolation without network calls.
- You can’t mock it without stubbing the module.
The Refactor: Inject Dependencies
function fetchUser(httpClient, userId) {
return httpClient.get(`/users/${userId}`);
}
const apiClient = axios.create();
fetchUser(apiClient, 42);
Why This Scales
- Swap any implementation (HTTP clients, services, mocks).
- Unit tests are trivial: pass a fake client.
- Clear ownership: each function declares what it depends on.
Pro Tip: Start with manual DI. If your system gets big, introduce a DI container (e.g., InversifyJS) to manage dependencies at scale.
2. Command Pattern: Stop Writing Giant Switch Statements
A growing system leads to feature sprawl.
Most devs respond by adding more cases to existing handlers until everything becomes a god function.
The Problem
function handleAction(actionType, payload) {
switch (actionType) {
case 'CREATE_USER':
// create logic
break;
case 'DELETE_USER':
// delete logic
break;
// more cases...
}
}
This breaks easily. Adding new actions increases cognitive load and risk.
The Refactor: Command Objects
Each action is its own object with a shared interface.
class CreateUser {
execute(payload) {
// create user logic
}
}
class DeleteUser {
execute(payload) {
// delete user logic
}
}
const commands = {
CREATE_USER: new CreateUser(),
DELETE_USER: new DeleteUser(),
};
commands[actionType].execute(payload);
Why This Scales
- Adding features doesn’t change old code.
- Each command has one responsibility.
- Easy to unit test commands in isolation.
- Can evolve into CQRS, job queues, or task scheduling as needed.
Pro Tip: Wrap commands in pipelines if you need consistent behaviors like validation, authorization, or logging.
3. Pure Function Extraction: Make Your Logic Testable and Predictable
Stateful code doesn’t scale.
When you’re not sure where side effects happen, you’ll waste time debugging.
The Problem
function updateUserProfile(user) {
user.name = formatName(user.name);
user.lastUpdated = new Date();
saveToDB(user);
}
- It mutates
user
in place. - You don’t know where the data flows.
- You can’t reuse this logic in pure functional pipelines.
The Refactor: Pure Functions
Separate data transformation from side effects.
function normalizeUserProfile(user) {
return {
...user,
name: formatName(user.name),
lastUpdated: new Date(),
};
}
Why This Scales
- Pure functions are predictable.
- Side effects are contained and obvious.
- You can compose transformations without breaking behavior.
- Testing pure functions is trivial: input → output, no mocking.
Pro Tip: Enforce immutability with tools like Immer or Immutable.js if you’re working on complex, nested data.
4. Event Emitter Pattern: Decouple Your System
Direct calls between modules create tight coupling.
Tight coupling does not scale.
The Problem
function createUser(user) {
saveUser(user);
sendWelcomeEmail(user);
addToCRM(user);
}
This works until you need to:
- Add more listeners.
- Handle failures in one listener without breaking others.
- Scale across services or processes.
The Refactor: Event Emitters
import EventEmitter from 'events';
const events = new EventEmitter();
events.on('user.created', sendWelcomeEmail);
events.on('user.created', addToCRM);
function createUser(user) {
saveUser(user);
events.emit('user.created', user);
}
Why This Scales
- You decouple producer and consumers.
- You can add/remove listeners without breaking code.
- Easy to fan out into queues (RabbitMQ, Kafka) later.
- Works for in-process, inter-process, or distributed event-driven systems.
Pro Tip: Use a dedicated event bus abstraction instead of raw EventEmitter
. Even better, standardize payloads with schemas for validation.
5. Factory Functions: Composition Over Inheritance
Classes encourage inheritance.
Inheritance doesn’t scale.
Composition does.
The Problem
class User {
constructor(name) {
this.name = name;
}
sayHello() {
return `Hi, I'm ${this.name}`;
}
}
this
context is fragile.- Inheritance chains grow and break.
- Harder to compose multiple behaviors cleanly.
The Refactor: Factory Functions
function createUser(name) {
const sayHello = () => `Hi, I'm ${name}`;
return { name, sayHello };
}
const user = createUser('Matcha');
user.sayHello(); // Hi, I'm Matcha
Why This Scales
- No
this
context issues. - Easy to compose multiple factories.
- Functions return plain objects—simple to test and use.
- No inheritance, no surprises.
Pro Tip: Factories work beautifully with dependency injection. Return pure objects or closures that expose clean APIs.
TL;DR
Refactoring isn’t cleanup. It’s architecture.
You’re laying the foundation for:
✅ Faster development cycles
✅ Stable feature growth
✅ Easier onboarding of new devs
✅ Lower risk of regressions
Use These 5 Patterns:
- Dependency Injection → decouple and test better
- Command Pattern → isolate behavior and scale features
- Pure Function Extraction → isolate logic and predict behavior
- Event Emitter Pattern → decouple producers from consumers
- Factory Functions → favor composition over inheritance
🔐 Premium Subscriber Exclusive:
Get the JavaScript Refactoring Cheat Sheets & Blueprints—a hands-on guide to scaling your codebase using framework-agnostic patterns.
📥 Download the full resource here: