Learning React isn’t hard — but sometimes you still need that reference sheet training wheel to help you along until you get the hang of balancing yourself on the code.
Here is a quick summary/notes on React components and classes. In short — basically, everything you sort of need to know/be aware of to get started with React classes.
** the information found here can also be found on React’s documentation. This post is more a summary of the documentation, with a few additional notes. Also, hope you like my attempts at making a summary/sharable infographic.
JavaScript Notes for React
- React tend to define their variables with
let
andconst
instead ofvar
. - React uses the
class
keyword but has its own ‘flavor’ of it. You don’t need to put commas between class method definitions and the value ofthis
depends on how it is called. - Arrow functions
=>
are preferred over regular function syntax. Arrow functions also don’t have their ownthis
value, which is good for preserving thethis
value from an outer method definition.
React Components & Props
- React is used to describe what the UI should look like.
- JSX is a template language with JavaScript abilities
- React is focused on separation of concerns based on feature and functionality rather than the file type.
Here’s an example diagram borrowed from one of my previous posts on SoC:
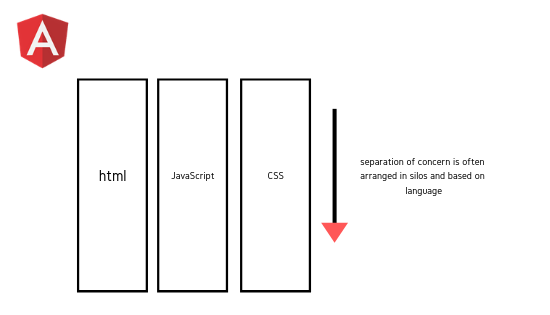
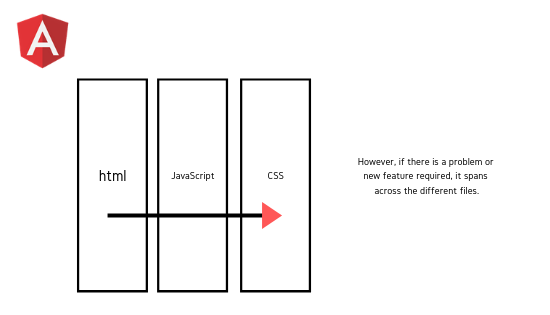
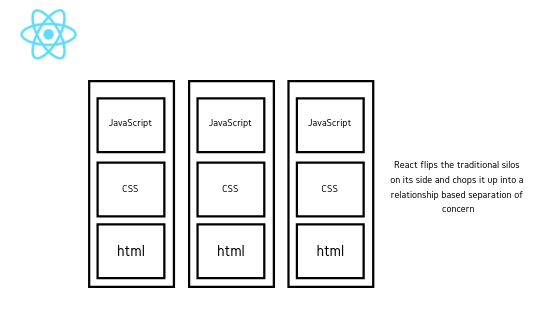
- each ‘unit’ of code is called a
component
. - each
component
is expected to be independent, reusable, and can exist in isolation. - inputs are called
props
.props
are object arguments and is read-only. Modifying aprop
is an anti-pattern. You can do whatever you want with theprop
— just don’t change the original value. Clone it. Copy it. Duplicate it. Just don’t change it.
Code syntax for React component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
React class component syntax:
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Rendering components:
const element = <Welcome name="Tibbers" />;
ReactDOM.render(
element,
document.getElementById('root')
);
Multiple/composing components:
function App() {
return (
<div>
<Welcome name="Tibbers" />
<Welcome name="Teemo" />
<Welcome name="Gertrude" />
</div>
);
}
ReactDOM.render(
<App />,
document.getElementById('root')
);
Here is the summary for this section.
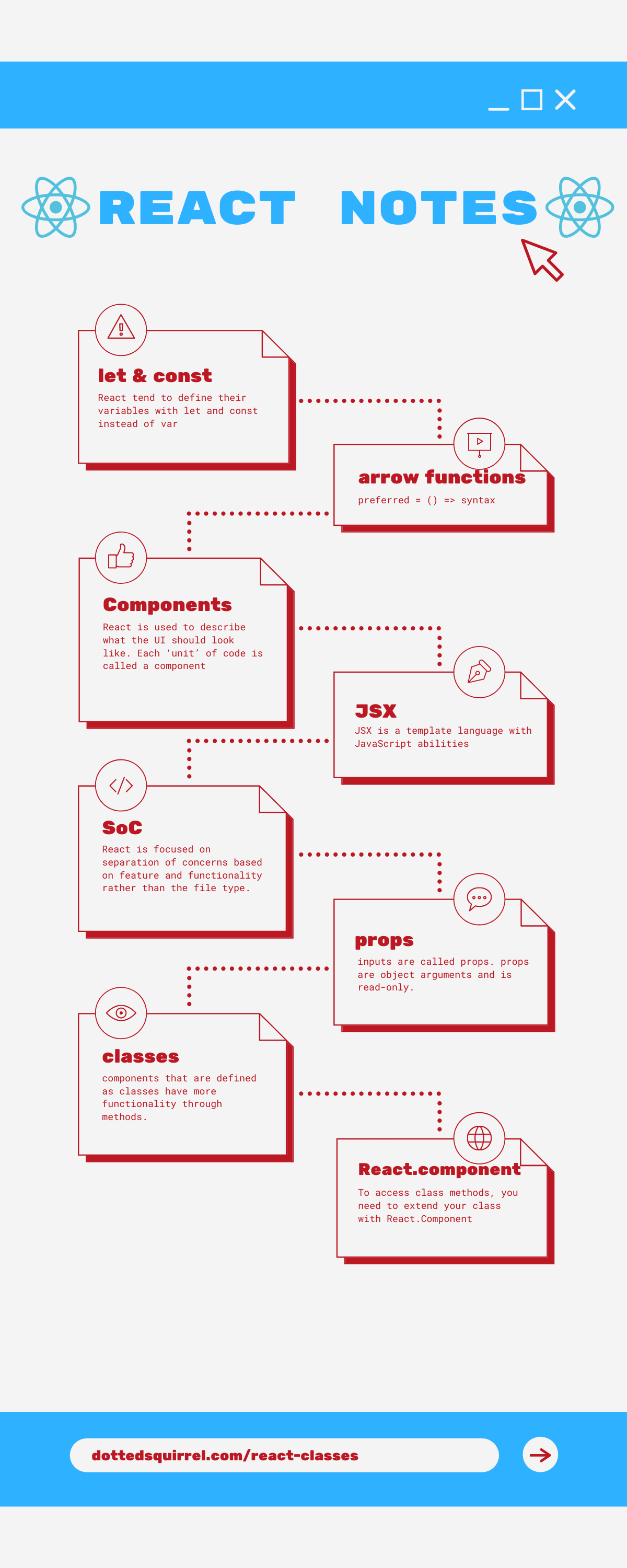
React classes
- React
components
that are defined asclasses
have more functionality through methods. - To access
class methods
, you need toextend
yourclass
withReact.Component
. render()
is the only compulsory method inReact.Component
- lifecycle methods are methods that you can use at a particular stage in the component’s lifecycle. Here is a diagram:
Here is the summary of the above and a general overview of what’s about to follow.
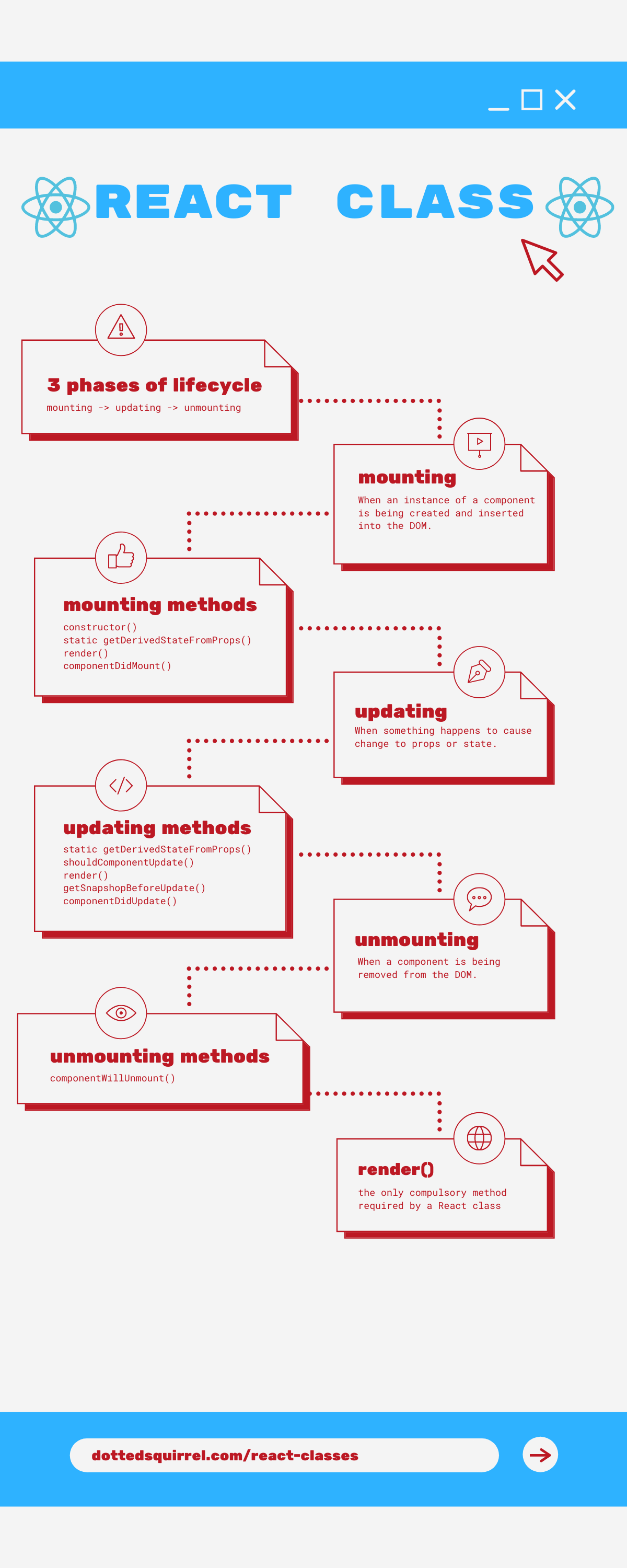
List of React lifecycle phases, methods available, and summary of what they do
React Lifecycle: Mounting
When an instance of a component is being created and inserted into the DOM. Here are the methods available in this phase and what they do.
constructor()
- if you don’t need states or bind methods, you don’t need a constructor.
- constructors in React are called before it gets everything else and
super(props)
needs to come before any other statement orthis.props
will beundefined
. - the point of having constructors is to initialize local state by assigning an object to
this.state
and binding event handler methods to an instance
Here’s what constructor()
looks like:
constructor(props){
super(props);
//assinging the initial state
this.state = {name: 'Tibbers' };
}
static getDerivedStateFromProps()
- called before
render()
- for usage when state depends on changes in props over time
- not really used because it’s expensive to process because it gets fired on every render, regardless of trigger or cause
- here’s an article from React on why you shouldn’t use it in general
render()
- the only required method for a React class component
- can return the following: React element (e.g.
<MyComponent />
), arrays and fragments, portals (for rendering your children into different DOM subtree), string and numbers, booleans or null (which renders nothing) render()
function shouldn’t modify component state and just return things. i.e. all logical processing should exist outside ofrender()
componentDidMount()
- invoked when a component is inserted in the DOM tree (i.e. mounted). Any initialization such as loading data from remote endpoints and setting up subscriptions should go here
setState()
can be called incomponentDidMount()
. This will trigger an extra round of rendering but before the browser shows anything on the screen. This is good if you’re waiting for things to arrive via API — but can cause performance issues because you’re rendering the view twice.
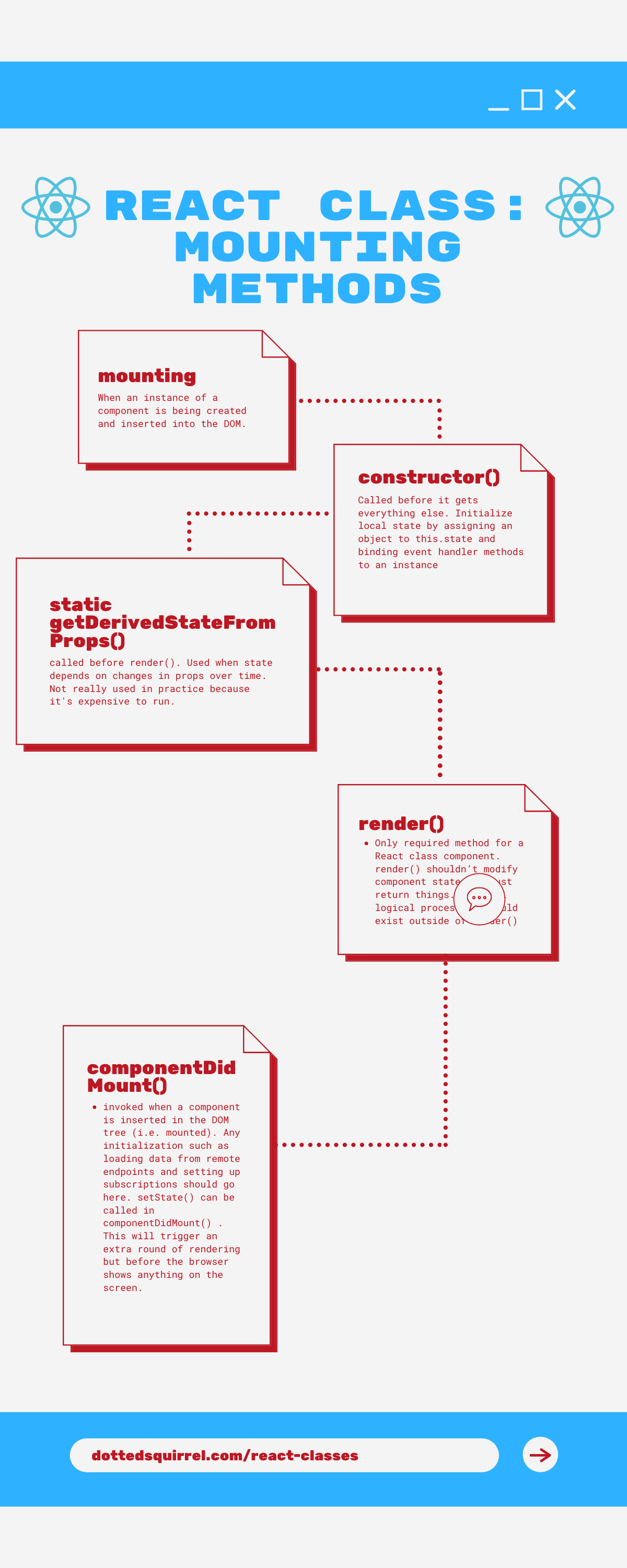
React Lifecycle: Updating
When something happens to cause change to props or state. Here are the methods available in this phase and what they do.
static getDerivedStateFromProps()
- gets invoked right before
render()
gets called - used for returning an object to update the state, or
null
to update nothing - not often used
shouldComponentUpdate()
- invoked before new props or state is recieved
- checks if a component’s output is not affected by the current change
- another rarely used lifecycle method and is used for performance optimization, but doesn’t actually prevent rendering itself. It returns
true
orfalse
depending on the component’s output and its relation to change
render()
- the only required method in a React class
getSnapshotBeforeUpdate()
- used to capture information from the DOM before it is potentially changed, for example, a scroll position
- snapshot value or
null
expected as a return
componentDidUpdate(preProps)
- invoked immediately after updating occurs. Not called after the initial render.
setState()
can be called insidecomponentDidUpdate()
but it needs to be wrapped in a condition or you will cause an infinite loop
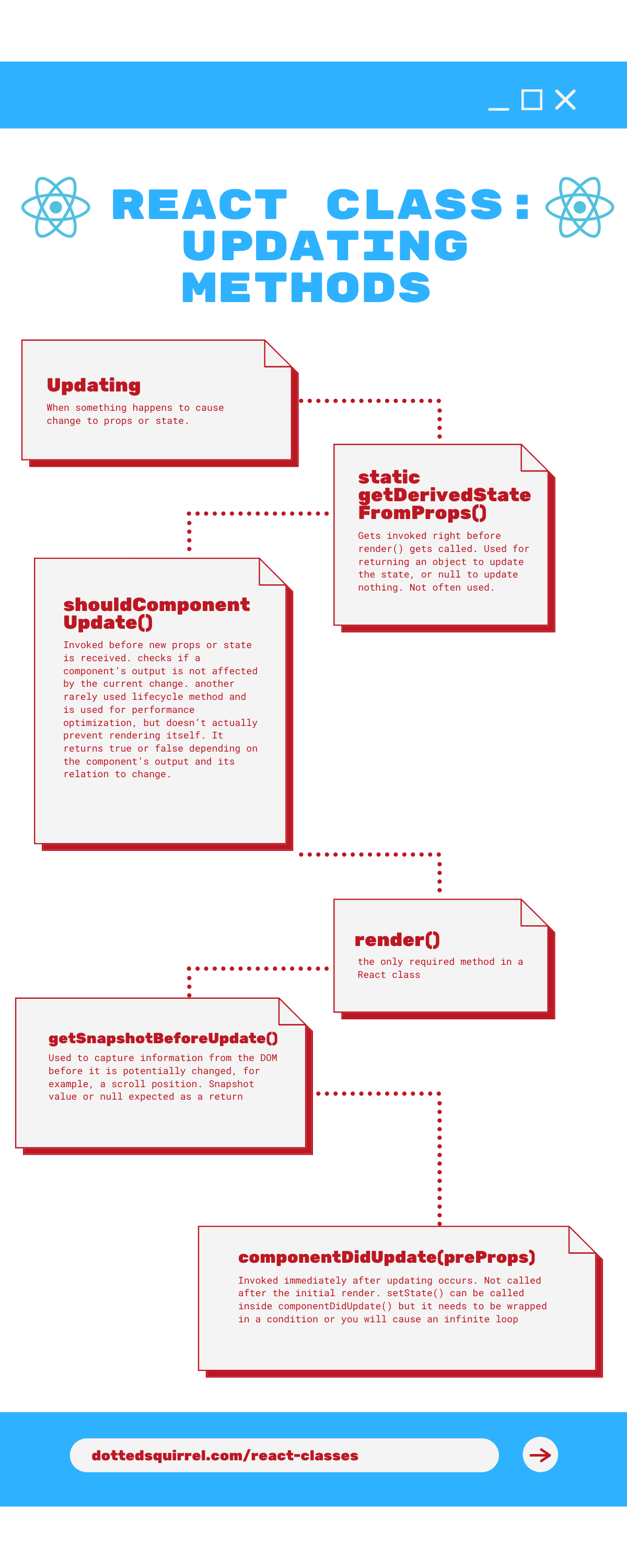
React Lifecycle: Unmounting
When a component is being removed from the DOM. Here are the methods available in this phase and what they do.
componentWillUnmount()
- invoked before component is unmounted and destroyed. Good place to clean up connections like network requests, or any subscriptions created in
componentDidMount()
- not a good place to call
setState()
because your component is about to be destroyed and once it gets unmounted, everything is lost.
Wrap up: Where to from here?
React classes is only one section of React. Other spaces to explore include but is not limited to are:
- handling events
- conditional rendering
- lists and keys
- forms
- lifting states
- composition vs. inheritance
Depending on how this piece is received, I might turn this into a series and do more notes style posts on the above topics. Hope you’ve found the info here useful and thank you for reading. 👏😜🙃🤪