Front end technology, especially JavaScript, has historically been looked down upon by back end veterans as not being a proper language. Mostly because JavaScript started off as function based language — making it immature in the ways of potential growth and what it can do.
I remember back in the late 90s and early 2000s when JavaScript was mainly used for making html pages slightly more dynamic. Sometimes it would put a few visual features here and there.
Nowadays, there are entire frameworks, libraries and even back end systems running on JavaScript. Making a native mobile and desktop app with JavaScript was unheard off — but nowadays, it’s as common, if not more popular, than Java itself — complete with cross platform support.
JavaScript is everywhere. It’s easy to get your hands dirty with JavaScript and make something useful with it. But with ease comes potential long term issues. A lot of JavaScript programmers aren’t trained in the ways of object oriented programming. It’s not their fault, not really. Sometimes, we just get caught up in trying to get things work. Sometimes, we just don’t know what we don’t know.
What is object oriented programming?
Object oriented programming is based on a different mentality. The idea behind it is that you create a blueprint for what your ‘object’ looks like and call that over and over again to do whatever you want with it.
Each time you want to use an object, you have to create it first so it exists, then set its properties in order to use the functionalities attached to it. These functionalities are known as ‘methods’.
For example, a customer order object might have a get order details functionality (aka method) attached to it.

In a functions based structure, you will need to inject a dependency into the function to make it work. Perhaps you’ll need to figure out what the order id is and inject it into the function.
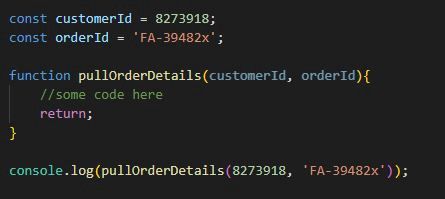
The issue with the above becomes that if you were to expand the number of functions, this can soon get quite messy. While it seems initially much easier to just write everything as functions and call it as needed, the lack of scope definition can lead to a chaining effect that impacts on a number of unknown but connected relationships. Classes, however prevent this.
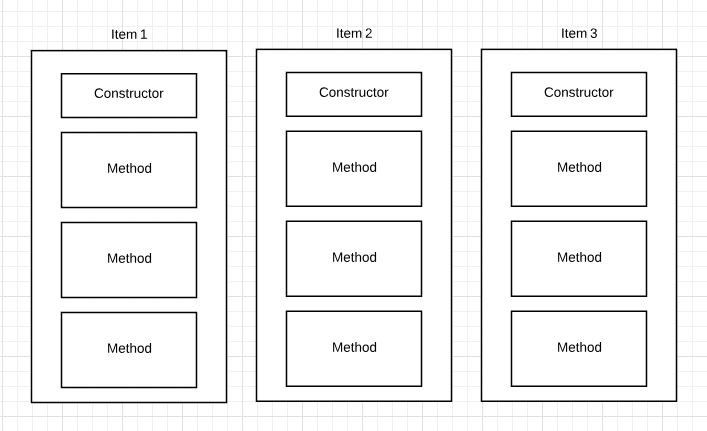
The constructor is the place where variables are set. Getter and setter methods are entry points into the class that does something. What functions are used and how they are used are hidden. Each time a new object is created, the entire class and its methods are ‘cloned’ and become accessible to that particular item. The scope of change is defined and we know that any change to a class and its methods will be uniformed.

When we code with a bunch of loose functions, its scope of change is not often defined. Dependency injection is required to make the function work and one function often requires another function to work. On face value, functions based programming may seem easy at first but in the long run, it is a logical nightmare to maintain.
With object oriented programming, you just need to call the getter and setter methods to access the black box of functionality. As a consumer of the class, you don’t need to know how it works. You just need to know that it works.
Why do we need to implement OOP in JavaScript?
Spaghetti code arises when there are too many things interconnected with each other. Functions based programming, which is what JavaScript is initially, may be quick to code but in the long term, it creates a high level of risk and multiple points of failure for the final application.
As the code base grows, you’re going to need to change the way you arrange your thoughts and start thinking in terms of objects. The scope of objects are easier to mentally grasp and trace than a series of interconnected functions that are strung together through a series of dependency injections.
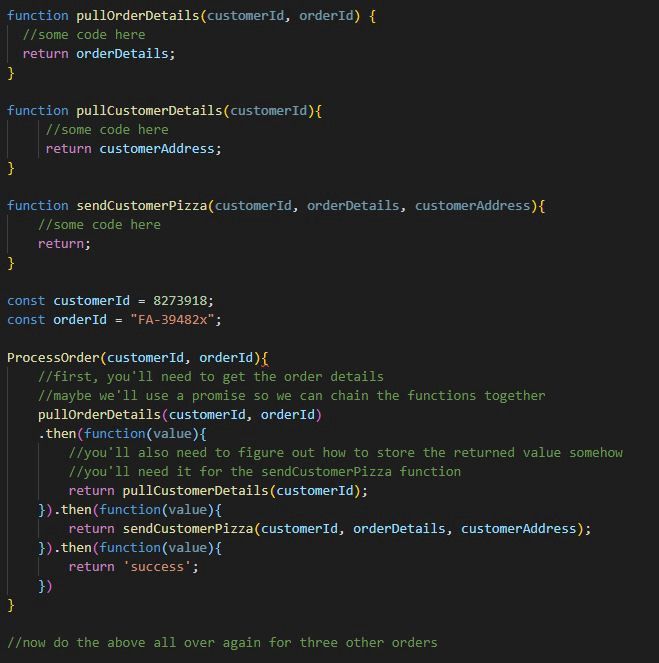
The issue with functions based programming is that a break in chain can result in the failure of the entire flow. With objects, one broken method doesn’t (and shouldn’t) impact on the rest of the class.
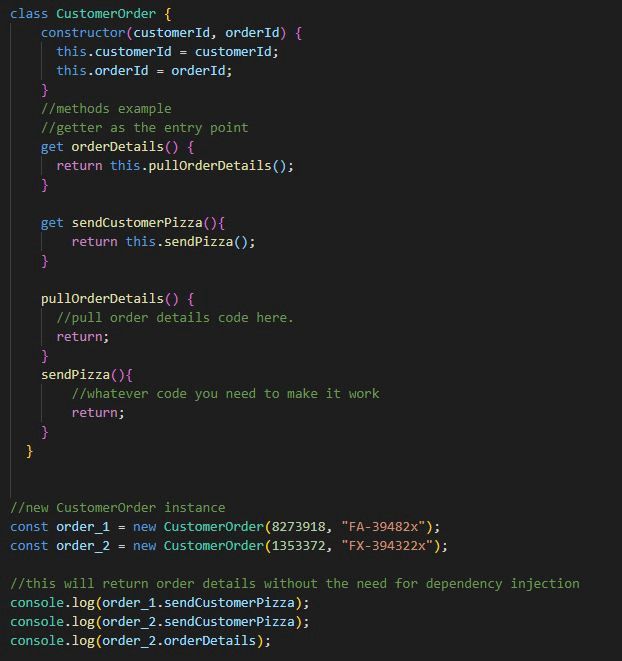
When you based your thinking around classes rather than a series of interconnected functions, you are reducing the risk and scope of failures if one arises. That’s because every dependency inject creates a potential point of failure. Not only is it a time cost to trace a series of functions, it’s an even bigger time and mental cost if you have to do it a dozen times for exactly the same thing.
Final Words
I often think of object oriented programming as the active decision to contain and ring fence the scope of whatever I’m working on. Angular requires an overall understanding of the framework in order to implement OOP — something which I think I’ll need to do in a completely separate post in the future.
Overall, OOP in JavaScript can lessen the mental load and potential spaghetti like relationships that is inherent in functions based programming. While it might have worked a few decades ago and in very small and single responsibility applications, the front end and JavaScript based back ends have grown both in size and complexity.
At the end of the day, all code is the same — just structured differently. The Object Oriented paradigm was thought up as a solution to naked functions based programming — which required a lot more mental load to figure out than a class based structure. When the structure of code is easily understandable and traceable, it reduces the chance of errors, making it easier to add new features without breaking everything else that surrounds it.