JavaScript Optional Chaining (?.) Explained with Code Examples (and Cheatsheet)
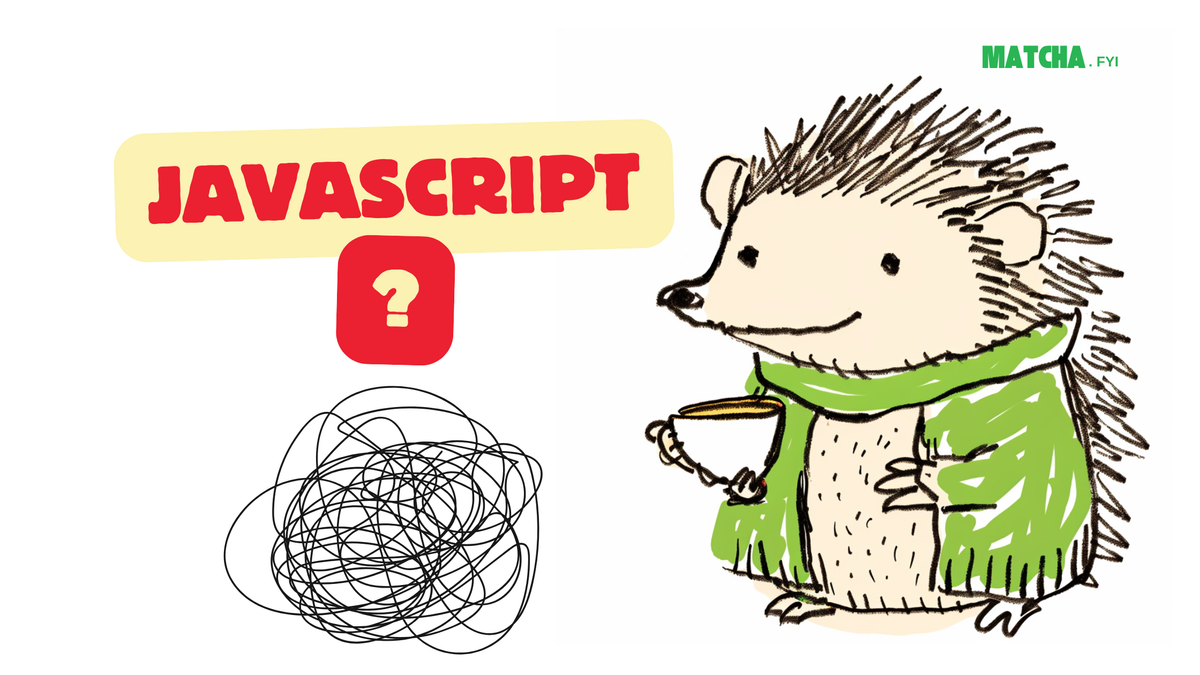
JavaScript Optional Chaining (?.
) is the cleanest way to avoid those frustrating runtime errors when accessing deeply nested properties in objects or arrays. If you've ever written endless if
checks or chained &&
operators to prevent your app from crashing, optional chaining is the upgrade you need.
This guide explains exactly how optional chaining works in JavaScript, with real-world examples you can copy and paste. Pro Members can grab an exclusive cheatsheet and a production-ready code template pack at the end.
What is Optional Chaining (?.
) in JavaScript?
Optional Chaining is an operator in JavaScript (?.
) that allows you to safely access nested object properties, array items, or even call functions—without throwing an error if an intermediate value is null
or undefined
.
Quick Example
Without Optional Chaining
const userCity = user && user.address && user.address.city;
With Optional Chaining
const userCity = user?.address?.city;
If user
or address
is undefined
or null
, JavaScript returns undefined
instead of throwing an error.
How to Use Optional Chaining in JavaScript (With Code Examples)
1. Accessing Nested Object Properties
const user = {
name: "Alice",
address: {
city: "Wonderland"
}
};
const city = user?.address?.city;
console.log(city); // "Wonderland"
const zip = user?.address?.zip;
console.log(zip); // undefined (no error)
2. Accessing Array Items Safely
const users = [
{ name: "Alice" },
null,
{ name: "Bob" }
];
const secondUserName = users?.[1]?.name;
console.log(secondUserName); // undefined (no error)
3. Calling Functions Safely
const user = {
getName: () => "Alice"
};
const name = user.getName?.();
console.log(name); // "Alice"
const userWithoutGetName = {};
const missingName = userWithoutGetName.getName?.();
console.log(missingName); // undefined (no error)
Optional Chaining Syntax (?.
) Breakdown
Use Case | Syntax | Example |
---|---|---|
Property Access | object?.property | user?.address?.city |
Array Access | array?.[index] | users?.[1]?.name |
Function Call | object?.method?.() | user.getName?.() |
Common Mistakes with Optional Chaining
1. Only Works on undefined
or null
Optional chaining does not skip over other falsy values like false
, 0
, NaN
, or an empty string ""
.
const obj = { flag: false };
const flag = obj?.flag;
console.log(flag); // false (works as expected)
2. Overusing Optional Chaining
Avoid slapping ?.
everywhere. It’s a tool for safe access, not a substitute for solid data validation or clean structures.
Why Use JavaScript Optional Chaining?
- Avoid Runtime Errors: Say goodbye to
Cannot read property
errors. - Cleaner Code: No more bloated
if
statements. - Better Readability: Code is more declarative and easier to scan.
- Modern JavaScript: Supported in modern browsers and Node.js 14+.
FAQs About JavaScript Optional Chaining
What is JavaScript Optional Chaining?
Optional Chaining (?.
) allows safe access to nested properties in JavaScript without throwing an error if a reference is null
or undefined
.
How does the optional chaining operator (?.
) work?
It checks if the object is null
or undefined
before attempting to access the next property, element, or call a function.
When should you use optional chaining?
When accessing properties or calling methods on objects that might be missing or undefined, such as API responses or dynamic data.
Why You Should Start Using JavaScript Optional Chaining
Optional chaining simplifies your JavaScript code and makes it safer to access deeply nested data. Now that you know how it works, add it to your toolkit.
🔐 Pro Cheatsheet for Optional Chaining (?.
)
Everything you need to avoid undefined
errors and write clean, modern JavaScript—plus bonus code snippets for React, Node, and APIs.
📥 Download the cheat sheet here: