Clean Code Is Killing JavaScript Performance
Clean code looks great in reviews—but it's killing your JavaScript performance. Over-abstraction, closures, and DRY dogma are slowing down your apps. Sometimes, the fastest code is the ugliest.
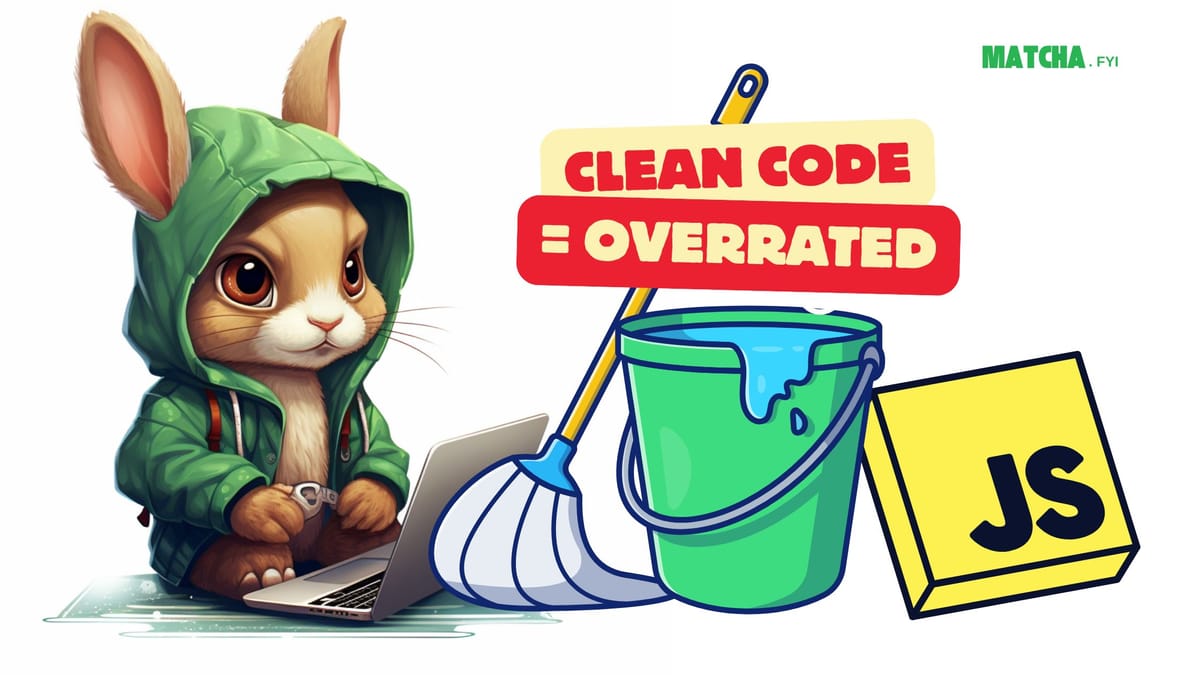
There’s an uncomfortable truth JavaScript developers don’t want to hear: your obsession with “clean code” is slowing down your applications. That perfectly abstracted, elegantly named, lovingly formatted codebase? It’s a performance dumpster fire.
We’ve spent years glorifying clean code principles—SRP, DRY, KISS, SOLID. Don’t get me wrong, these ideas are useful in moderation. But the cult of clean code has pushed JavaScript devs to write software that prioritizes aesthetics over speed. And users are paying the price.
Readability Over Reality
You create a simple function. It works. Then you think, "Hmm, let’s abstract that out." Then you do it again. And again. Suddenly, you have a hundred tiny, beautifully named functions, all calling each other in a cascading waterfall of inefficiency. This happens all the time in codebases worshipping "clean code" principles.
Consider a typical "clean" approach to calculating a cart total:
function calculateTotal(cartItems) {
return cartItems.reduce((total, item) => total + getItemPrice(item), 0);
}
function getItemPrice(item) {
return item.quantity * getUnitPrice(item);
}
function getUnitPrice(item) {
return item.price - getDiscount(item);
}
function getDiscount(item) {
return item.onSale ? item.price * 0.1 : 0;
}
Looks clean. It’s easy to follow in a code review. But the runtime tells a different story. On large datasets, this introduces hundreds—if not thousands—of unnecessary function calls. JavaScript engines like V8 have gotten better at optimizing functions, but they can't eliminate the overhead entirely.
Now compare it to this version:
function calculateTotal(cartItems) {
let total = 0;
for (let i = 0; i < cartItems.length; i++) {
const item = cartItems[i];
const discount = item.onSale ? item.price * 0.1 : 0;
const unitPrice = item.price - discount;
total += item.quantity * unitPrice;
}
return total;
}
No indirection. No overhead. Just raw, fast iteration. This version can be up to 30% faster on large arrays, depending on the browser engine. Yes, it’s less "clean." But users don’t care about how elegant your abstractions are. They care about speed.
Abstraction as a Performance Killer
Modern JavaScript engines are optimized for predictable code paths and tight loops. Excessive abstraction breaks both. Every level of indirection makes it harder for the engine to inline functions, optimize hidden classes, and reduce memory churn.
For example, closures—while powerful—introduce complexity for the garbage collector:
function createIncrementer(start) {
return function increment() {
return ++start;
};
}
const inc = createIncrementer(0);
Each closure holds onto its surrounding scope. Multiply this by thousands of closures across components (hello, React hooks), and memory pressure skyrockets. V8’s garbage collector is good, but not magic. You will hit GC pauses, and they will tank your performance.
DRY Is a Trap (Especially in Hot Paths)
"Don’t Repeat Yourself" often leads to premature abstraction. Here’s a DRY version of a simple operation:
function multiplyByTwo(arr) {
return arr.map(double);
}
function double(num) {
return num * 2;
}
Versus the "dirty" way:
function multiplyByTwo(arr) {
let result = new Array(arr.length);
for (let i = 0; i < arr.length; i++) {
result[i] = arr[i] * 2;
}
return result;
}
The loop version eliminates callback allocation, function context switching, and leverages predictable memory allocation. This approach consistently benchmarks faster in tight loops—sometimes 50%+ faster on large datasets.
Garbage Collection Loves Clean Code (But That’s Bad)
In React, "clean" hooks encourage closures everywhere:
function App() {
const [count, setCount] = useState(0);
const increment = useCallback(() => setCount(count + 1), [count]);
return <button onClick={increment}>Increment</button>;
}
Unless you’re meticulously managing dependencies and memoization, closures are constantly reallocated on every render. In small apps, fine. At scale? You’re dragging GC along for the ride, introducing jank, render delays, and frame drops.
Optimized versions avoid unnecessary closures:
function App() {
const [count, setCount] = useState(0);
function increment() {
setCount(c => c + 1);
}
return <button onClick={increment}>Increment</button>;
}
No need for useCallback
here—just a stable function reference. Less GC overhead, fewer unnecessary re-renders.
Frameworks Make Clean Code Addictive (And Slow)
Component composition looks clean but adds real cost:
<ExpensiveComponent>
<AnotherComponent>
<YetAnotherComponent />
</AnotherComponent>
</ExpensiveComponent>
Each component has lifecycle hooks, reactivity, and rendering logic. Every layer introduces a potential render bottleneck. Framework devs will tell you to "memoize everything"—which often just adds more code complexity and more memory usage.
Sometimes the fastest solution is to flatten the tree and render directly. Yes, it’s uglier. Yes, it’s harder to reuse. But if you’re building for speed (think high-frequency trading dashboards, real-time gaming UIs), you have to make trade-offs.
Profile or You’re Guessing
Want to be useful? Stop debating clean code in code review. Open Chrome DevTools. Profile your hot paths. Look at CPU timelines. Analyze memory snapshots.
Example: Developers complained about jank in a React table component displaying 10,000 rows. Clean, composable row components. Memoized to death. Still laggy.
Solution? Ditch components. Render raw HTML inside a documentFragment
. Batch DOM updates. The table went from 2 seconds render time to 200ms. Ugly code. Users loved it.
Clean Code Is a Luxury You Might Not Afford
Performance-first coding isn’t clean. It’s calculated. It’s benchmark-driven. It’s ugly, but it works.
Clean code is killing JavaScript performance because we prioritize developer experience over user experience. If you care about speed, you need to be willing to write code that offends code reviewers and delights your users.
Write ugly code when it matters.
Clean code is killing JavaScript performance.
And it’s time we admit it.
Comments ()